This page is dedicated to the GPU support and documentation of the MAPSIM toolbox available at simtoolbox.github.io
The MapcoreCuda library is C++/CUDA extension to the MATLAB and can be used upon successfull compilation directly from the the Matlab’s command line interface. It supports both single and double precision computation on an arbitrary GPU card. Multiple GPU cards are not supported at this time (IE only the firsrt available is used). The library can be used on both Windows and Linux platforms. No other external dependencies are required except for the vendor (NVIDIA) provided libraries:
The average speedup of the computation will vary based on CPU/GPU used for the comparison as well as on the computing parameters, but is roughly 10-20x . In order to obtain the best performance results, always use a GPU with the newest PCIe specification support in order to improve data transfers between the CPU and GPU. At the time of 2024, most GPUs do support PCIe Gen. 4.0 (nvidia RTX 4000 series). The only supported GPUs are from nVidia. The library will not work on Intel / AMD and/or 3rd party GPU platforms.
The library has no limitations other than the physical GPU card itself (Most notable is the memory limit). Please note that double precision computing will usually incur a performance loss. For double precision computing, the nVidia Tesla series are recommended. Under most circumstances, the recommended data type to use is single precision (float). For maximum performance, please consider cropping the images so that each of the dimension’s size is equal to 2^N (For example 256 x 512 pixels) – this also applies to CPU version by the way. Amount of images is arbitrary.
Before Compilation
It is important to make sure that the library can be compiled for the GPU you are targeting. As the times go by, older GPU models and their architectures are slowly becomming obsolete. The first thing is therefore a crosscheck of the available GPU with the supported platform list by the CUDA SDK. Generally speaking, the Geforce / Quadro and Tesla GPU series are supported.
- Find the corresponding GPU in the database HERE and note its compute capability (CC).
- Check if the architecture is supported for compilation by the NVCC compiler HERE.
At the time of writing this post (2024) CUDA SDK 12.4 has support for nVidia Maxwell and newer GPU architectures (GTX 900 series onwards). Feel free to skip this check if your have a “new” machine. A great tool to identify you hardware is GPU-Z as shown below (Linux’s equivalent is the CPU-X application or gpustat for performance monitoring):
It is highly recommended to update your nVidia Drivers to the latest suppoorted version (This is done by the the CUDA SDK). Latest nVidia drivers are available for download HERE. The compilation process of the Mapsim acceleration library is 2-step, in the first step, the acceleration dynamic library ( MapcoreLib.dll for windows / MapcoreLib.so for linux) is build. In the second step, the corresponding matlab mex file is build (MapcoreCuda.mexw64 for windows/ MapcoreCuda.mexa64 for linux).
Windows Compilation
In order to compile the library under windows, the following prerequisites are required:
- Install the Microsoft Visual Studio with the Support for C++ compilation.
- Install the required CUDA SDK Version and update your drivers. Recommended is a clean install.
NOTE: You can have multiple CUDA SDK versions Installed at the same time. - Have a Matlab version of you choosing available and installed. No additional Matlab add-ons are required.
NOTE: Previous MATLAB versions do not need to be un-installed.
Make sure that the Visual Studio is supported in the CUDA SDK in the Installation instructions HERE (This might cause some problems only in case a newer MSVC has just been released a few months ago – in that case, consider installing rather the previous version). Once all prerequisities are installed, clone the library sources from git repository – these include the Microsoft Visual Studio solution file (.sln).
The easiest approach is to open both *.vcxproj files (MapcoreLib/MapcoreLib.vcxproj and MapcoreCuda/MapcoreCuda.vcxproj) files with a text editor and lookup the following information: [ ***CUDA 12.3.props*** and ***CUDA 12.3.targets***]. Change the values according to the CUDA SDK version installed or required (If the Appropriate SDK is not installed, then Visual Studio will complain). Then open the entire solution (.sln) and modify according to your needs the following settings for both projects (MapcoreCuda & MapcoreLib):
- MapcoreLib >> Properties >> Configuration Properties >> VC++ Directories >> Include Directories
Make sure to change to the Matlab installation path IE “C:\Program Files\MATLAB\R2023b\extern\include“ - MapcoreLib >> Properties >> Configuration Properties >> VC++ Directories >> Library Directories
Make sure to change to the Matlab installation path IE “C:\Program Files\MATLAB\R2023b\extern\lib\win64\microsoft“
For MapcoreLib, go to MapcoreLib >> Properties >> Configuration Properties >> CUDA C/C++ >> Device >> Code Generation and make sure that the compilation will be executed for the GPU architecture of your choosing. You can include just one or all of the options supported by NVCC. To see the supported options, open a windows command line and type in “nvcc –list-gpu-code” and “nvcc –list-gpu-arch“.At this point, the MapcoreLib project should compile just fine and the result would be a MapcoreLib.dll. In order to compile the Matlab Mex file and link it with the MapcoreLib library, the following Changes are required:
- MapcoreCuda >> Properties >> Configuration Properties >> VC++ Directories >> Include Directories
Include path to the folder containing the MapcoreLib source files (Mapcore.cuh etc.). - MapcoreCuda >> Properties >> Configuration Properties >> VC++ Directories >> Library Directories
Include path to the release folder of the MapcoreLib (Must contain MapcoreLib.lib and MapcoreLib.dll).
At this point, both MapcoreLib.dll and MapcoreCuda.mexw64 should build fine. These are the only 2 files required. If the system cannot find for whatever reason the cufft and cublas libraries, feel free to copy them to accessible location from “C:\Program Files\NVIDIA GPU Computing Toolkit\CUDA\v12.3\bin”. Again, only cublas*.dll | cufft*.dll and cudart*.dll are required.
Feel free to follow the Entire Microsoft Visual Studio Instructions on how to create the project from beginning.
Linux Compilation
In order to compile the sources under linux, none of the sln and vcxproj files are used. Instead 2 makefiles are used. The prerequisities will up to some point vary based on the linux distribution (Tested distribution is OpenSUSE 15.5), but mostly consists of the following items:
- Development tools for C/C++
See if you package manager offers installation on a pattern base.
For OpenSUSE, install the “Base Development” and “C/C++ Development” patterns through yast, which include GCC / GPP and make utilities, which are the bare minimum. For ubuntu/kubuntu, the corresponding equivalent should be package build-essential. Feel free to change the default OS compiler version to your needs OpenSUSE offers GCC 7 / 11 / 12 / 13 versions. Note that Matlab has also a supported compiler list, crosscheck the version HERE. As of 2024, the recommended version is GCC11. - CUDA SDK Installed accroding to the Linux Installation Guide for your distribution. Note that not all of the available distributions might be supported. Once the CUDA SDK is installed, do not forget to add the CUDA SDK to the PATH ( nano ~/.bashrc amd add line “export PATH=/usr/local/cuda-12.3/bin${PATH:+:${PATH}}”). The system should be able to recognize “nvcc” command thereafter.
- Installed Matlab version of your choosing. Follow the official Installation instructions for your distribution.
After all the prerequsities are installed, the compilation process is quite simple. Navigate via terminal to the MapcoreLib folder and execute “make“. The same applies for the MapcoreCuda – which must be compiled after successfull compilation of the MapcoreLib. To change the default compilation settings, open each of the makefiles with a text editor (nano / kate / code …) and verify/adjust the following variables:
- MATLABROOT (Installation path for Matlab)
- CUDA_SDK (Version of the CUDA SDK)
NOTE: It is expected that the default installation location is at “/usr/local/cuda*” - GPP_VER (Choosen G++ compiler version)
- Feel free to adjust the “Code Generation section” of the NVCC to match the GPU architecture of your need.
After both makefiles are executed, the Release folder should contain “MapcoreLib.so” and “MapcoreCuda.mexa64” which are the only required files. The dynamic library (.so), must be further accessible by matlab. As such, it is recommended to modify the LD_LIBRARY_PATH to include the file’s path. IE for example: (nano ~/.bashrc and add “export LD_LIBRARY_PATH=/WORK/GIT/mapsim/Releases”). Dont forget to start a new console session after modifying the bashrc file.
Changes from last version published in 2017:
-
-
- Improved performance
- Improved PCIe transfer speed
- Reduced GPU memory usage
- Refactored Codebase
- Added support for linux compilation
-
The Matlab-to GPU implementation will generally vary and doesnt include the same sort of methematical operations in the same sequence (IE is not 1:1). As such, the results from matlab and GPU will vary up to some extent. It is important to note that by default, the “use fast math” compilation switch is also used for GPU code compilation. This increases performance at a cost of precision. Feel free to match optimization goals to your needs though. The library is by default compiled for all supported GPU architectures by the CUDA SDK – IE the “code generation” section adjustments can be skipped. The following command shows how to use the library:
[Im_Map,Iter,Alpha,Thresh] = MapcoreCuda(Im_Seq,Im_Masks,OTF,MaxIter,Thresh,Lambda,Alpha)
For more information about the parameters and their function, visit the official MAPSIM documentation. Below are the precompiled files for Windows and Linux Platforms (Excluding the CuBLAS and CuFFT .dll libraries – these needs to be installed via the corresponding CUDA SDK by selecting to install CUDA Runtime as shown below – It is recommended to select the “network install” during download)
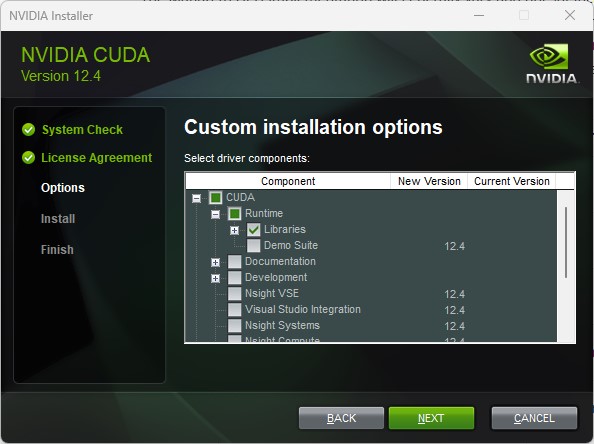
DOWNLOADS
For compatibility reasons, it is recommended to download version that matches the matlab version. Mixed versions might not work as documented HERE.